Controling a stepper motor with NodeJS
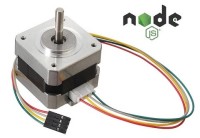
In this article i will detail how to use NodeJS to control a bipolar stepper motor.
In this project, I will describe a simple NodeJS script to control a stepper motor. For further information abaout NodeJS, Please refer to this previous article : https://www.elektormagazine.com/labs/i2c-communication-using-nodejs-and-raspberry-pi
The connection diagram is given in the file 'Stepper Schematic.pdf' (See attachments). As you can see we use a L298 H bridge controller board. This item can be bought at this address : https://www.aliexpress.com/item/1005002255724701.html?spm=a2g0o.productlist.0.0.49c9cf51gOay8N&algo_pvid=feff4fbc-f324-4faf-9c93-54a4fb4cc8fe&algo_exp_id=feff4fbc-f324-4faf-9c93-54a4fb4cc8fe-10
As usal, the HMI is a simple web page containing three elements :
This time we must transfer two information to the NodeJS script :
The difficult part is to create a correct 'get' request.. This is simply done by these lines of the HTML script :
The line below creates the request from two information :
So, if we enter 150 steps and check the option button 'Left', the request is : /go/150/Left
In NodeJS these information are processed by these lines :
The lines below describe how these information are used by NodeJS
The array 'Command' contains four sub-arrays containing the values to give to the inputs IN1, IN2, IN3 and IN4 of the control board. These values will be used to power the coils of the motor through the L298 driver.
The connection diagram is given in the file 'Stepper Schematic.pdf' (See attachments). As you can see we use a L298 H bridge controller board. This item can be bought at this address : https://www.aliexpress.com/item/1005002255724701.html?spm=a2g0o.productlist.0.0.49c9cf51gOay8N&algo_pvid=feff4fbc-f324-4faf-9c93-54a4fb4cc8fe&algo_exp_id=feff4fbc-f324-4faf-9c93-54a4fb4cc8fe-10
As usal, the HMI is a simple web page containing three elements :
- A Textbox in which the user will indicate the number of steps the axis of the motor will turn
- A group of Radio buttons with which the user will indicate The direction, clockwise or counter clockwise, the axis of the motor will turn
- A Button to start the rotation of the motor
This time we must transfer two information to the NodeJS script :
- The number of steps
- The direction in which the motor will rotate : Clockwise (Right) or Counter Clockwise (Left)
The difficult part is to create a correct 'get' request.. This is simply done by these lines of the HTML script :
var radios = document.getElementsByName("Direction"); var checked; if (radios[0].checked) { checked="Left"; } else { checked="Right"; } $.get('/go/' + document.getElementById("Text_Box").value + '/' + checked) .done(function(Donnee_Recue) { $('#message').html(Donnee_Recue); });First we retrieve which radio button is checked
The line below creates the request from two information :
- The number of steps written in the Textbox
- The direction retreived from the Radio button group
$.get('/go/' + document.getElementById("Text_Box").value + '/' + checked)
So, if we enter 150 steps and check the option button 'Left', the request is : /go/150/Left
In NodeJS these information are processed by these lines :
Server.get('/go/:ns/:d', function(request, respons) { N_Steps=parseInt(request.params.ns,10); Direction=request.params.d;
- First line, the server receives the 'get' request, in which 'ns' and 'd' are two parameters corresponding to number of steps and direction. Note these parameters are indicated with ':'.
- Second line: The parameter 'ns' is extracted (" request.params.ns"), converted into Int value and affected the the variable N_Steps.
- Third line : The parameter 'd' is extracted (" request.params.d") and affected the the variable Direction
The lines below describe how these information are used by NodeJS
The array 'Command' contains four sub-arrays containing the values to give to the inputs IN1, IN2, IN3 and IN4 of the control board. These values will be used to power the coils of the motor through the L298 driver.
//IN1 IN3 IN2 IN4 var Command=[[1,1,0,0],[0,1,1,0],[0,0,1,1],[1,0,0,1]]; if(Direction=="Left") { for(a=0; a Why do we use the remainder of a division by 4 ? The answer is in the document 'stepper-command.png' which explains the command sequence of a bipolar stepper motor. For example, if we command the 17th step, the remainder of 17/4 is 1. In this case we use the sub-array [0,1,1,0] and the logic levels applied on the inputs are :
- IN1 = Low
- IN2 = High
- IN3 = High
- IN4 = Low
Now, if we command the 18th step , the remainder of 18/4 is 2. In this case we use the sub-array [0,0,1,1] and the logic levels applied on the inputs are : - IN3 = Low
- IN4 = High
And so on, and so on...
Enjoy !
:-))
Discussion (1 comment)