Digital Music box
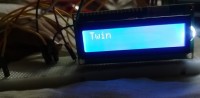
This digital music box plays a tune and shows the lyrics in an LCD monitor.
What is a music box?
A music box is a small musical instrument. It can play a particular Tune.
It consists of some metal teeth arranged in a row.
The music box has a handle. With each rotation of the handle, each of the teeth moves one by one and produces a musical note. After the movement of all the teeth, a full song/music is played.
Today we will make an Arduino-based Digital Music Box. It will have a push-button, an LCD, and a Buzzer. With each press of the button, one musical note will be played in the buzzer and you’ll see the corresponding lyric on the LCD. We will play the tune ‘Twinkle twinkle little star’ in our music box.
Components:
Arduino UNO R3 x 1
Active Buzzer x 1
Push button x 1
10K volume POT x1
Breadboard x 1
16x2 LCD with header x 1
Jumper wires x 1
10K resistor x 1
Connection:
| Arduino UNO | Buzzer
| 9 | +
| GND | -
| Arduino UNO- R3 | LCD
| GND| VSS,K, 1st pin of volume POT
| 5V | VDD,A, 2nd pin of volume POT
| | V0, 3rd pin (wiper) of the volume POT
| GND | RW
| 12 | RS
| 11 | E
| 5,4,3,2 | D4,D5,D6,D7
Code:
A music box is a small musical instrument. It can play a particular Tune.
It consists of some metal teeth arranged in a row.
The music box has a handle. With each rotation of the handle, each of the teeth moves one by one and produces a musical note. After the movement of all the teeth, a full song/music is played.
Today we will make an Arduino-based Digital Music Box. It will have a push-button, an LCD, and a Buzzer. With each press of the button, one musical note will be played in the buzzer and you’ll see the corresponding lyric on the LCD. We will play the tune ‘Twinkle twinkle little star’ in our music box.
Components:
Arduino UNO R3 x 1
Active Buzzer x 1
Push button x 1
10K volume POT x1
Breadboard x 1
16x2 LCD with header x 1
Jumper wires x 1
10K resistor x 1
Connection:
| Arduino UNO | Buzzer
| 9 | +
| GND | -
| Arduino UNO- R3 | LCD
| GND| VSS,K, 1st pin of volume POT
| 5V | VDD,A, 2nd pin of volume POT
| | V0, 3rd pin (wiper) of the volume POT
| GND | RW
| 12 | RS
| 11 | E
| 5,4,3,2 | D4,D5,D6,D7
Code:
//To know details about musical notes, frequency and Period, please visit http://www.arduino.cc/en/Tutorial/melody #include // initialize the library by associating any needed LCD interface pin // with the arduino pin number it is connected to const int rs = 12, en = 11, d4 = 5, d5 = 4, d6 = 3, d7 = 2; LiquidCrystal lcd(rs, en, d4, d5, d6, d7); int buzzerpin = 9;//Buzzer is connected to Digital Pin 9 int buttonpin=8; char notes[] = "ccggaagffeeddcggffeedggffeedccggaagffeeddc"; // a space represents a rest int beats[] = { 1, 1, 1, 1, 1, 1, 2, 1, 1, 1, 1, 1, 1, 2, 4,1,1,1,1,1,1,1,1,1,1,1,1,1, 1, 1, 1, 1, 1, 2, 1, 1, 1, 1, 1, 1, 2, 4 }; char lcd_text[42][10]={"Twin","kle","twin","kle", "lit","tle","star","How","I","won","der","what","you", "are","up","a","bove","the","world","so","high", "like","a","dia","mond","in","the","sky","Twin","kle","twin","kle", "lit","tle","star","How","I","won","der","what","you", "are"}; int tempo = 300; int buttonpress=0; int numberofpress=0; boolean oldbuttonState = LOW; boolean newbuttonState = LOW; void playTone(int tone, int duration) { for (long i = 0; i < duration * 1000L; i += tone * 2) {//timeHigh = period / 2 = 1 / (2 * toneFrequency// digitalWrite(buzzerpin, HIGH);// make buzzerpin high delayMicroseconds(tone); //wait for tone microseconds digitalWrite(buzzerpin, LOW);//Make buzzerpin low delayMicroseconds(tone); //Wait for tone microseconds } } void playNote(char note, int duration) { char names[] = { 'c', 'd', 'e', 'f', 'g', 'a', 'b', 'C' }; //Notes int tones[] = { 1915, 1700, 1519, 1432, 1275, 1136, 1014, 956 };//High time, Time period,T=1/f, frequency=f, Time period/2=High time // play the tone corresponding to the note name for (int i = 0; i < 8; i++) { if (names[i] == note) { playTone(tones[i], duration); } } } void setup() { pinMode(buzzerpin, OUTPUT); pinMode(buttonpin,INPUT); Serial.begin(9600); lcd.begin(16, 2); } void loop() { newbuttonState=digitalRead(buttonpin); if (newbuttonState != oldbuttonState) { if (newbuttonState==HIGH) { delay(200); numberofpress++; if(numberofpress==43) { numberofpress=1; } Serial.print(numberofpress); playNote(notes[numberofpress-1], beats[numberofpress-1] * tempo); delay(tempo /2); lcd.clear(); lcd.print(lcd_text[numberofpress-1]); } } oldbuttonState= newbuttonState; }
Discussion (1 comment)