Wireless IoT modules from Pycom
LoPy - LoRa
As far as I know there is no LoRa gateway where I am doing these tests, and even if there would be I wouldn’t know how to connect to it, but it is possible to make two LoPy modules talk to each other without the need of a gateway, so let’s try that instead. You will need, of course, two LoPy modules…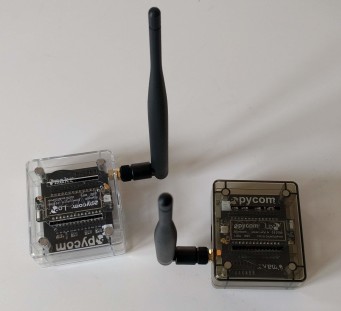
After flashing the latest firmware on the boards I put the following code in the file main.py on board 'A' (using my FTP client):
from network import LoRa
import socket
import time
import pycom
lora = LoRa(mode=LoRa.LORA, frequency=863000000)
s = socket.socket(socket.AF_LORA, socket.SOCK_RAW)
s.setblocking(False)
pycom.heartbeat(False)
while True:
if s.recv(64) == b'Ping':
pycom.rgbled(0x007f00) # green
time.sleep(0.05)
pycom.rgbled(0) # off
s.send('Pong')
time.sleep(2.5)
pycom.rgbled(0x7f0000) # red
time.sleep(0.05)
pycom.rgbled(0) # off
time.sleep(2.5)
This way board 'A' will flash red every five seconds. When it receives the word ‘Ping’ it will flash green too.On board B I added the code below to main.py to make board B send the word ‘Ping’ every five seconds and flash green. It does not check for any incoming data.
from network import LoRa
import socket
import time
import pycom
lora = LoRa(mode=LoRa.LORA, frequency=863000000)
s = socket.socket(socket.AF_LORA, socket.SOCK_RAW)
s.setblocking(False)
pycom.heartbeat(False)
while True:
s.send('Ping')
pycom.rgbled(0x007f00) # green
time.sleep(0.05)
pycom.rgbled(0x000000) # off
time.sleep(5)
This worked fine. Since there is apparently no addressing involved this seems to be some sort of broadcast setup meaning that it should be possible to add more LoPy boards to the network.Read full article
Hide full article
Discussion (0 comments)